# Orders
Including
application/json
in the Accept header will return the Order as a JSON, otherwise the Order will be in a signed JWT.
Whenever a
verification required
error is returned, 3D Secure needs to be performed.
# Create an order with a card
When a cardholder enters their card number as a one time event, a card token is created which can be used to create an order.
To create an order, post an Order Creatable
to the order endpoint. The payment
field must be a Card Payment Creatable
.
# Request
POST /v1/order
Host: merchant.intergiro.com
Content-Type: application/json
Accept: application/json
Authorization: Bearer <customer.api.key> | Bearer <private.api.key>
{
"items": <number or item information or array of items objects>,
"currency": "<currency of the transaction>",
"charge": "auto", // optional
"payment": {
"type": "card"
"card": "<card token>"
"client": {
"browser": // <Browser object>
}
}
}
}
See browser
section for information on how to get the browser information above.
# Response
On success, the response will be an Order
, otherwise an Error
will be returned together with the id of the order.
IMPORTANT: Include the
id
from the error response in the request body for all following calls to the order-create endpoint, concerning the same order.** This is important to keep a correct History of the authorization creation, and to make sure 3DS is done correctly. The id field in an Order Creatable should never be populated with any id other than the id received from the order endpoint.
# Customer initiated order with a saved card
# 1. Perform 3DS and update the payment method
When a customer makes a payment with a saved card, 3DS authentication is required.
To create an order with a selected Payment Method, the payment method needs to be converted into a Card Payment Creatable. For this, and to perform 3DS, the <intergiro-payment-update>
component can be used. More information and full example can be found here.
# 2. Use the updated payment method to create a new order
After 3DS has been performed and payment method updated, payment can be made.
POST an Order Creatable
with
- the payment returned from the submit function of the
<intergiro-payment-update>
component populated on thepayment
field, - the customer ID populated on the
customer
field
POST /v1/order
Host: merchant.intergiro.com
Content-Type: application/json
Accept: application/json
Authorization: Bearer <customer.api.key> | Bearer <private.api.key>
{
"items": 20,
"currency": "EUR",
"customer": "TjbHYXXn6yEdhLgS",
"payment": {
"card": "<card.token>",
"type": "card",
"subsequent": { "reference": "MCA1400001202", "initiator": "cardholder" }
}
}
# Merchant initiated order for an existing customer
To create a customer order post an Order Creatable
to the order endpoint,
the customer
field must be set to a customer id and the payment
field must be a Customer Payment Creatable
.
# Request
POST /v1/order
Host: merchant.intergiro.com
Content-Type: application/json
Authorization: Bearer <customer.api.key> | Bearer <private.api.key>
{
"number": <your order identifier>,
"items": <number or item information or array of items objects>,
"customer": <customer id>,
"currency": <currency of the transaction>,
"payment": {
"type": "customer"
}
}
# Response
{
"id": "<Identifier of order in Intergiro's system>",
"created": "<datetime of order>",
"customer": "<id of customer or contact information>",
"items": "<numer or item information or array of items objects>",
"currency": "<Currency of order>",
"payment": "<Card payment>",
}
# Create an order with a payment link
To create an order with a payment link, make a request to the order endpoint with an Order Creatable
as body.
The payment needs to be of type Defer Payment Creatable
as in the below example.
The response will include a payment link which will redirect the customer to a checkout page where they can enter their card details and pay for the order.
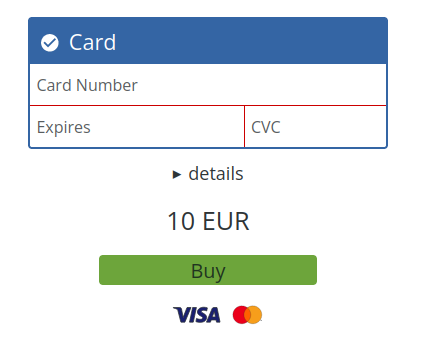
- To include a field for the customer to enter the cardholder name, set
payment.cardholderName
to "required". - A notification can be received when the customer has paid the order, by specifying a callback URL in the request, see Callbacks.
- To redirect the customer to a specific URL after completing the payment process, assign the URL to the
payment.redirectUrl
field.
# Request
POST /v1/order
Host: merchant.intergiro.com
Content-Type: application/json
Accept: application/json
Authorization: Bearer <customer.api.key> | Bearer <private.api.key>
{
"number": "<your order identifier>",
"items": <number or item information or array of items objects>,
"currency": "<currency of the transaction>",
"charge": "auto",
"payment": {
"type": "defer",
"method": "link",
"cardholderName": "required",
"redirectUrl": "<payment completion redirect URL>"
}
}
# Response
{
"id": "<Identifier of order in Intergiro's system>",
"created": "<datetime of order>",
"items":"<numer or item information or array of items objects>",,
"currency": "<Currency of order>",
"charge": "auto",
"payment": {
"type": "defer",
"method": "link",
"created": "<datetime of payment>",
"status": "created",
"service": "defer",
"amount": "<Amount of payment>",
"currency": "<Currency of payment>",
"link": "<Link to payment>",
},
"event":"<Order events>",
"status": "<Status of order>"
}
# Change an order
In order to capture, refund or cancel an order you must change it.
To change an order make a PATCH containing an array where each element contains an id of the order and an array of Event Creatable
for the changes you want to apply on given order.
# Request
PATCH /v1/order
Host: merchant.intergiro.com
Content-Type: application/json
Authorization: Bearer <private.api.key>
[
{
"id": "<Identifier of order in Intergiro's system>",
"event": [<Array of Event Creatables>]
},
...
]
# Response
You should get a 200 response with the same changes you requested in the body.
[
{
"id": "<Identifier of order in Intergiro's system>",
"event": [<Array of Event Creatables>]
},
...
]
# List all orders
To list the orders send a GET request to the order endpoint. It is highly recommended to use the date
queries when listing orders, to optimize behaviour.
# Request
GET /v1/order?start=2000-01-01&end=2000-02-01
Host: merchant.intergiro.com
Content-Type: application/json
Authorization: Bearer <customer.api.key> | Bearer <private.api.key>
# Response
The response will be an array of orders
.
# Callbacks
A callback URL can be added to the order to get notified when the order has been updated, such as being charged, refunded or canceled. Add a callback
property to the Order Creatable
when creating the Order, specified with the URL you wish to receive the notification.
You will receive the order as a signed JWT (content-type: application/jwt
), and the order will be updated with the successful or failed Event
.
# Verifying the Order JWT
After receiving an Order as a JWT, you may optionally choose to verify it.
Verifying the order returns the full order details and ensures that it is originating from Intergiro.
To verify, use the /worker-order/order/verify
endpoint with the signed JWT as the body.
# Request
POST /v1/worker-order/order/verify
Host: merchant.intergiro.com
Content-Type: application/jwt
Authorization: Bearer <private.api.key> or <public.api.key>
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6IjFOQk9xaWNzZUhZTmhhQk4iLCJtZXJjaGFudCI6InRlc3R.....
# Response
{
"id": "<Identifier of order in Intergiro's system>",
"number": "<Identifier optionally provided by merchant>",
"created": "<datetime of order>",
"customer": "<id of customer or contact information>",
"callback": "<callback url>",
"items": "<order amount or item information>",
"currency": "<Currency of order>",
"payment": "<Card payment>",
"status": "<Order status>",
"event": [List of Order Events]
}
← Styling Customer API →